![[Singleton pattern]](/2017/11/25/Singleton/singleton1.png)
Sometimes we need to make sure that we have only one instance of an object throughout the entire life-cycle of an application. There may be many reasons for that, but one of the most commons is to avoid overuse of computer resources.
In the definition of the singleton design pattern there are two major points,
- There can only be one instance of an object at any given time.
- We should allow global point of access to that single instance.
Singletons are very easy to understand, however when it comes to implementation several concerns start to appear. Imagine we are in a multithreaded environment and two different threads request instantiation at the same time. Although this may seem like a long shot, the true is that it happens and will probably break the application.
What if someone decides to use reflection to access the Singleton’s private constructor ?
This two points (and some others as Cloning or Serializing) have made the Singleton a controversial topic.
So let’s dig a little this Singleton thing,
How to instantiate a Singleton ?
There are two ways to instantiate a Singleton,
- Lazy instantiation
- Eager instantiation
Let’s see what this means,
In eager instantiation, the singleton instance is created as soon as the JVM does the classload. In other words, even if we are not yet using the singleton, the resources that the object eventually needs are already being consumed.
On the other hand, lazy instantiation will only consume the resources when we actually instantiate the singleton for the first time.
Although lazy instantiation is preferred we need to take some additional care if we decide to go for it, as while the eager instantiation is thread safe the lazy instantiation is not, and we will need to ensure it get’s synchronized.
We’re in Java..can we use an Enum as a Singleton ?
As Obama would say, Yes We Can.
In Java, it happens that Enums are singletons by nature and are globally accessible, so that makes them a good candidate when we want to use a Singleton, however there are some drawbacks in terms of flexibility and instantiation will always be eager.
How to represent a Singleton in UML ?
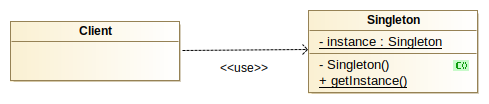
Client code requests an instance of the singleton object by calling the getInstance() method.
Note that Singleton constructor is private(-), and this will immediately invalidate the standard instantiation. Singleton singleon = new Singleton()
will not compile.
How do we test for Singletons ?
In Java we know that if two objects have the same hash code then they refer to the same object, so we can use that to test if our code is doing what is supposed to do.
Should we use Singletons ?
This is where the controversial starts…
My thoughts on singletons are quite practical. It depends.
Nowadays, we have lots of IOT products with little computing power, little resources and several controllers. We need to communicate with these hardware controllers in the most efficient way in order to save resources and optimize speed. So Singletons may very well be suited for the situation.
Never despise a programming technique, just because some people say it’s bad
(actually you may find people that say that all design patterns are bad in general !!).
Always give a little thought on the problem. Does it really need to be global ? Can we do it better ?
If you opt to go for the singleton, and that will become problematic at a later stage…well, you can be sure that you will know it. :)
In following articles we will visit some examples of the singleton pattern. You can follow the links bellow.