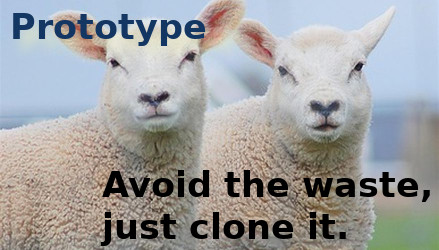
Whenever object instantiation implies a high computational cost or an uncertain availability of data, we should consider the possibility of cloning, as it may be cheaper than creating brand new instances.
Let us imagine an object that, while being initialized, calls a remote server to get some data for some of it’s attributes. Let’s also imagine that particular data changes only once every day at exactly midnight. Finally let’s pretend our system, needs to create one of those objects for each user and has an average of five hundred users per minute.
When we imagine a situation as described, some challenges immediately come up.
- We will need to make a call to a remote server around five hundred times per minute, which will increase the traffic on our network and put some strain in the database.
- What if the server on the other side does not respond ?
In a situation like this, instantiating objects can either become expensive (1), or become impossible, if the data source becomes unavailable (2).
This is where the prototype pattern comes in. We instantiate one object, store that object, and re-use it by making copies of it, keeping the original untouched.
Java actually made it very simple by providing us the Cloneable interface.
Is that all ?
Yes and No. When our object contains other objects inside, creating a copy of it has a pitfall we need to account for: we may need to clone the object and also all the objects inside. This introduces us to the concepts of shallow copy and deep copy and depending on our objective, we need to choose which method we want to implement.
Shallow copy
Shallow copy is the standard way in Java when we use the Cloneable interface. In a shallow copy we will receive a new object which is a precise copy of the original. This means that if an object has other objects inside, when we change one of the inner objects all copies (clones) will be affected.
in other words, the cloned object is a new object, but inner objects of the copy are exactly the same as in the original.
Deep copy
Deep copy method will provide us with a new object(the clone), maintaining the structure of the original object replicating it, but all inner objects will also be new. In this case, all inner objects will be independent, so any changes on them will only affect the particular object being altered and not all of them.
Obviously that deep copy involves a higher cost, as it has to instantiate all the inner objects of the original as well as copy their exact states, so depending on what we want to achieve we should dedicate some time to examine the situation.
In this article we will use an example that relies on primitives, so shallow copy will work perfectly.
The example
We will be creating a board game. For our board game we have three heroes (an Elf, a Wizard, and a Goblin). We will pretend that to instantiate our heroes, we need to read some values from a database as health, magic, attack and defense values. If our board is initialized with 10 of each hero, we would need to fetch values from the database several times…not a good idea…So we clone.
A simple UML representation of the prototype pattern for our case could be
![[click to full size]](/2017/12/22/Prototype-Pattern/Prototype.png)
In the above representation, Hero is the interface that will act as an contract for all heroes. Goblin, Elf and Wizard are the classes that define them, and we have a HeroWareHouse, that will be used to store one hero of each. Those will be our “master” copy for each one (remember that we are pretending that for each of them we are reading values from a database)
We will be using an UUID object to identify each hero. Some attention will be needed on that attribute, as each hero, will need to have a different UUID.
We will be using a support enumerator, which will act as the database for the heroes properties.
1 | package Prototype.Shallow; |
The Hero Interface, will extend Cloneable from the JAVA API. We could actually implement the Cloneable interface directly in each of the heroes classes but would make our code more complicated as having a common type to handle our Heroes simplifies our work.
1 | package Prototype.Shallow; |
Now we have the three Hero classes. In our little example they are very similar. For simplicity we harcoded the health value as 100 for all of them.
In the Hero classes we override the clone method, and please do note, that due the implementation we’ve used, we do need to cast the cloned object to the proper type before returning it.
In the event that our object can’t be cloned, we will return a null object. Although returning nulls is never a good solution, and could be swapped by the Java Optional, we will leave it as is for the sake of simplicity, and we do not even check for nulls later…(I, know..don’t crucify me)
1 | package Prototype.Shallow; |
The Goblin class definition
1 | package Prototype.Shallow; |
and finally the wizard
1 | package Prototype.Shallow; |
With the above in place, we go to the HeroWareHouse, which will act as a container of the Heroes “embryos”.
The warehouse is a simple hash map, and as we will only be storing one hero of each, the class name is used as the key. Not very sophisticated, but it does serves the purpose. :)
1 | package Prototype.Shallow; |
The game board will be a 20 x 20 grid represented by a two-dimensional array. Whenever an Hero is added a new UUID for that hero will be generated. A simple while loop is used to ensure that the square is empty, so that no Hero is overwritten.
1 | package Prototype.Shallow; |
So a little app to test all this
1 | package Prototype.Shallow; |
As said earlier in this article, we are pretending that heroes values are being fetched from a database. So, we instantiate just one of each hero (Elf,Goblin and wizard), and store one copy of each in the heroWarehouse.
Then after instantiating the game board, we fetch the heroes from the warehouse, and clone them, thus avoiding to repeat the process of fetching values from the database(here an imaginary one)
We will come back to the prototype pattern, in another article with an example of deep copy cloning.
An example for the code in this article can be found in git-hub