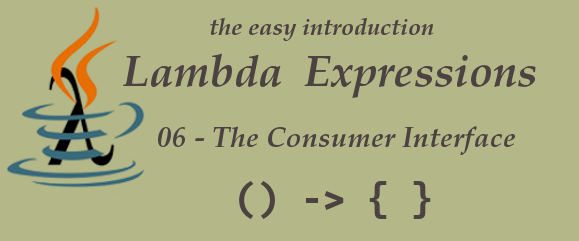
“Whatever goes in, never comes out” could be the Hollywood movie line to define a consumer, which is no more than a function that receives an argument, consumes it and returns nothing.
In this article I will talk about the Consumer functional Interface. Being a functional interface means it can be used as target type for lambda expressions.
A consumer is quite easy to explain. It is used whenever we need an object to be consumed. Consumed in this context means that a function receives an object as an input argument, eventually applies some kind of operation to that object, but nothing is returned from that function. In this cases, we say that the object has been consumed.
Let’s take a quick look to the Consumer interface source code,
1 |
|
The abstract method accept(), is responsible for receiving the object, performing whatever operation is defined in the lambda expression, and returns no value.
The andThen() method is a little more trickier. When applied, it returns a new consumer which represents the aggregation of both operations defined in both consumers.
We can write a quick example to demonstate the Consumer accept()
1 | public class ConsumerExampleOne { |
in the above example, the Consumer, is passed to the print() method together with an Integer and then just print’s the value.
We can also observe the behaviour of the andThen() method from the Consumer Interface, which combines two expressions,
1 | public class ConsumerExampleTwo { |
When run the above code will print,
consumer One :al
consumer Two :AL
consumer One :bc
consumer Two :BC
consumer One :cs
consumer Two :CS
consumer One :dr
consumer Two :DR
consumer One :ft
consumer Two :FT
Two consumers are created, consumer1 and consumer2. Both are dispatched to the print() method where they are combined originating the combinedConsumer reference. When the the accept() method is called on the combinedConsumer both operations defined in the consumer1 and consumer2 are applied.
A better example than just print something
As mentioned above, a consumer, can apply any transformation we want to an object. In the next example, we simulate a bank that charges a service fee every time a customer makes a deposit with a value lower than 200. We can use a combination of a predicate and a consumer to handle the job,
the Account class
1 | public class Account { |
The AccountProcessor Class
1 | import java.util.function.Consumer; |
And the App that will do some work,
1 | import java.util.function.Consumer; |
When run it prints,
No deposit Fee applied
Deposit Fee applied to account Number 2
Deposit Fee applied to account Number 3
201.0
177.5
77.5
Most of the above example is code just to make the simulation run. The relevant stuff for the example is the lowDepositServiceFee Consumer and the isLowDeposit Predicate references that are created in line 12 and 13 and later passed to the accountProcessor.deposit() method. This is where the predicate and the consumer do their magic in line 7 and line 8. Obviously this only works as an example, so if you do own a bank do not use this, as we are not even checking if the deposit is not negative !!! :)
Also note that in line 18, instead of passing the isLowDeposit reference, we passed the lambda expression directly, just to show that it also can be done in that way.
In the next post I will talk about the Supplier interface.
‘till then happy coding.